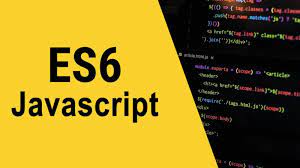
ES6 Features
In this Blog ,we will discuss some of the best and most popular ES6 features that we can use in everyday javaScript coding. ES6 is the 6th and major edition of the ECMAScript language specification standard. It defines the standard of the implementation of javascript and has become more popular than the previous edition ES5.
Some Of the ES6 features are:
- let and const Keywords
- Arrow Functions
- Template Literals
- Rest Parameter
- Classes
- Modules
- Spread operator
- Default Parameters
- Destructuring
1: let and const keyword:
-ES6 introduces the new let and Const keyword for declaring variables.Prior to ES6,the only
Way to declare a variable in Javascript was the Var keyword.
-Variables declared with let and const block-scoped, which means they are only accessible
within the block where they were declared. So, if we declare a variable with let, it does not
change its value in the outer scope.Constis similar, but the value of variables declared with this
keyword cannot change through reassignment.
// using `let`
let x = “global”;
if (x === “global”) {
let x = “block-scoped”;
console.log(x);
// expected output: block-scoped
}
console.log(x); // expected output: global
// using var:
var y = “global”;
if (y === “global”)
{
var y= “block-scoped”;
console.log(y);
// expected output: block-scoped
}
console.log(y); // expected output: block-scoped
2: Arrow Functions:
-Arrow functions allow a short syntax for writing function expressions. You don’t need the function keyword, the return keyword, and the curly brackets. example:
const x = (x, y) => x * y;
-Arrow functions do not have their own this. They are not well suited for defining object methods. Arrow functions are not hoisted. They must be defined before they are used.
3: Template Literals :
Template Literal in ES6 provides new features to create a string that gives more control over dynamic strings.Traditionally, String is created using single quotes (‘) or double quotes (“) quotes. Template literal is created using the backtick (`) character.
Syntax: var s=`some string`;
Multiline Strings: In-order to create a multiline string an escape sequence \n was used to give a new line character. However, in Template Literals there is no need to add \n string ends only when it gets backtick (`) character.
// Without template literal
console.log(‘Some text that I want \non two lines!’);
// With template literal
console.log(`Some text that I want on two lines!`);
4: Rest Parameter:
-Rest parameter is an improved way to handle function parameters, allowing us to more easily handle various inputs as parameters in a function. The rest parameter syntax allows us to represent an indefinite number of arguments as an array. With the help of a rest parameter a function can be called with any number of arguments, no matter how it was defined.
Syntax:
function functionname(…parameters) //… is the rest parameter (triple dots)
{
statement;
}
Example:
function fun(…input){
let sum = 0;
for(let i of input){
sum+=i;
}
return sum;
}
console.log(fun(1,2)); //3
console.log(fun(1,2,3)); //6
console.log(fun(1,2,3,4,5)); //15
5: Classes:
-In ECMAScript 5 and earlier, classes never existed in JavaScript. Classes are introduced in ES6 which look similar to classes in other object oriented languages, such as Java, PHP, etc., however they do not work exactly the same way. ES6 classes make it easier to create objects, implement inheritance by using the extends keyword, and reuse the code.
-In ES6 you can declare a class using the new class keyword followed by a class-name. By convention class names are written in TitleCase (i.e. capitalizing the first letter of each word).
class Rectangle {
// Class constructor
constructor(length, width) {
this.length = length;
this.width = width;
}
// Class method
getArea() {
return this.length * this.width;
}
}
// Square class inherits from the Rectangle class
class Square extends Rectangle {
// Child class constructor
constructor(length) {
// Call parent’s constructor
super(length, length);
}
// Child class method
getPerimeter() {
return 2 * (this.length + this.width);
}
}
let rectangle = new Rectangle(5, 10);
alert(rectangle.getArea()); // 50
let square = new Square(5);
alert(square.getArea()); // 25
alert(square.getPerimeter()); // 20
alert(square instanceof Square); // true
alert(square instanceof Rectangle); // true
alert(rectangle instanceof Square); // false
In the above example, the Square class inherits from Rectangle using the extends keyword. Classes that inherit from other classes are referred to as derived classes or child classes.
Also, you must call super() in the child class constructor before accessing the context (this). For instance, if you omit the super() and call the getArea() method on a square object it will result in an error, since the getArea() method requires access to this keyword.
6: Modules:
A module organizes a related set of JavaScript code. A module can contain variables and functions. A module is nothing more than a chunk of JavaScript code written in a file. By default, variables and functions of a module are not available for use. Variables and functions within a module should be exported so that they can be accessed from within other files. Modules in
ES6 work only in strict mode. This means variables or functions declared in a module will not be accessible globally.
Exporting a Module : The export keyword can be used to export components in a module.
Syntax:
export default component_name
export {component1,component2,….,componentN}
Importing a Module: To be able to consume a module, use the import keyword. A module can have multiple import statements.
Syntax:
import any_variable_name from module_name
import {component1,component2..componentN} from module_name
Example:
Step 1 ? Create a file company1.js and add the following code ?
let company = “TutorialsPoint”
let getCompany = function(){
return company.toUpperCase()
}
let setCompany = function(newValue){
company = newValue
}
export {company,getCompany,setCompany}
Step 2 ? Create a file company2.js. This file consumes components defined in the company1.js file. Use any of the following approaches to import the module.
import {company,getCompany} from ‘./company1.js’
console.log(company)
console.log(getCompany())
7: Spread Operator:
-JavaScript ES6 (ECMAScript 6) introduced the spread operator. The syntax is three dots(…) followed by the array (or iterable*). It expands the array into individual elements. So, it can be used to expand the array in a places where zero or more elements are expected
1. Copying an array
let fruits = [‘Apple’,’Orange’,’Banana’];
let newFruitArray = […fruits];
console.log(copiedList);
// [‘Apple’,’Orange’,’Banana’]
2. Concatenating arrays:
let arr1 = [‘A’, ‘B’, ‘C’];
let arr2 = [‘X’, ‘Y’, ‘Z’];
let result = […arr1, …arr2];
console.log(result);
// [‘A’, ‘B’, ‘C’, ‘X’, ‘Y’, ‘Z’]
3. Spreading elements together with an individual element
let fruits = [‘Apple’,’Orange’,’Banana’];
let newFruits = [‘Cherry’, …names];console.log(newFruits); // [‘Cherry’, ‘Apple’,’Orange’,’Banana’]
8: Default Parameter:
Now, in ES6 you can specify default values to the function parameters. This means that if no arguments are provided to function when it is called these default parameters values will be used. This is one of the most awaited features in JavaScript.
Example:
function sayHello(name) {
var name = name || ‘World’;
return ‘Hello ‘ + name + ‘!’;
}
console.log(sayHello());
// Hello World!
console.log(sayHello(‘John’)); // Hello John!
9:Destructuring:
–The destructuring assignment is an expression that makes it easy to extract values from arrays, or properties from objects, into distinct variables by providing a shorter syntax.There are two kinds of destructuring assignment expressions—the array and object destructuring assignment. Well, let’s see how each of them exactly works.
Example: Array Destructuring
let fruits = [“Apple”, “Banana”];
let [a, b] = fruits; // Array destructuring assignment
alert(a); // Apple
alert(b); // Banana
Example 2: Object Destructuring
et person = {name: “Peter”, age: 28};
let {name, age} = person; // Object destructuring assignment
alert(name); // Peter
alert(age); // 28
Referances: