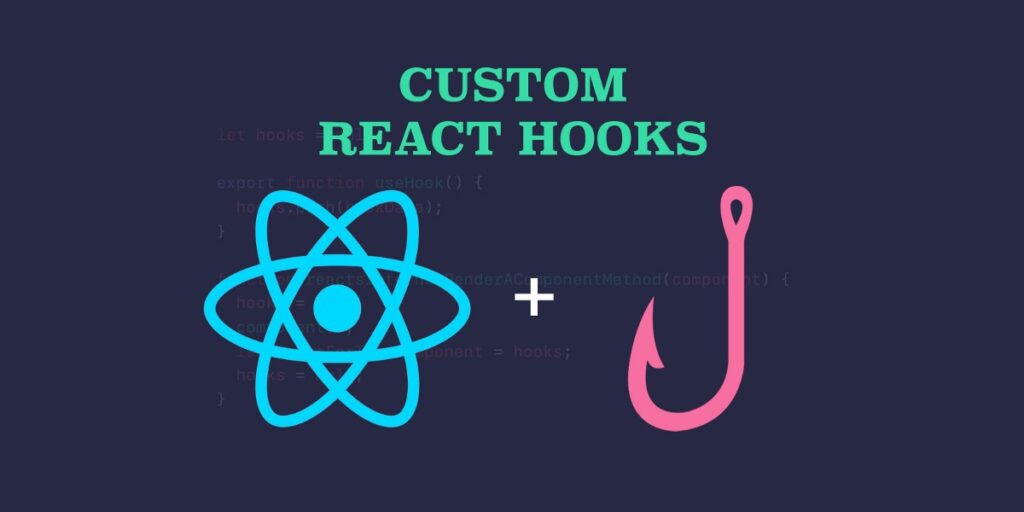
What is a Custom hook?
There might be instances where you have been using the same repetitive and redundant stateful logic inside multiple components. We were able to handle this situation by relying on Render props and Higher-Order Components. But with hooks, we can do it in a much simpler and cleaner way.
What are they?
These are normal javascript functions that can use other hooks inside of it and contain a common stateful logic that can be reused within multiple components. These functions are prefixed with the word “use”.
Rules of React Hooks:
- Only call Hooks at the top level. Don’t call Hooks inside loops, conditions, or nested functions
- Only call Hooks from React function components
- Don’t call Hooks from regular JavaScript functions (There is just one other valid place to call Hooks — your own custom Hooks. We’ll learn about them in a moment)
Example:
- We have two components, one is Employee Component and the other is Department Component. Employee Component connects to a Web API, fetches the employee data when the Component is mounted, and displays the data.
- Similarly, Department Component connects to a Web API, fetches the department’s data when the Component is mounted and displays the data.
- We might write the same code in both the Components to achieve this We were able to handle this by creating Render props and Higher-Order Components in the case of Class Components..
Custom Hook use list():
- Let’s create a function called as use list and this function accepts Web API URL as a parameter,
- Now let’s create a state variable in which we will store the list data and we will initialize it to an empty array.
- Now we will call the API within useEffect hook. I have the code handy and pasting it here.
- Now we will return the list from this function. Remember that it is a Javascript function and we can return anything as we want.
- Within that Javascript function, as we are using other React hooks then that function will be referred to as Custom Hook. Now we can call this hook from both Employee Component and Department Component.
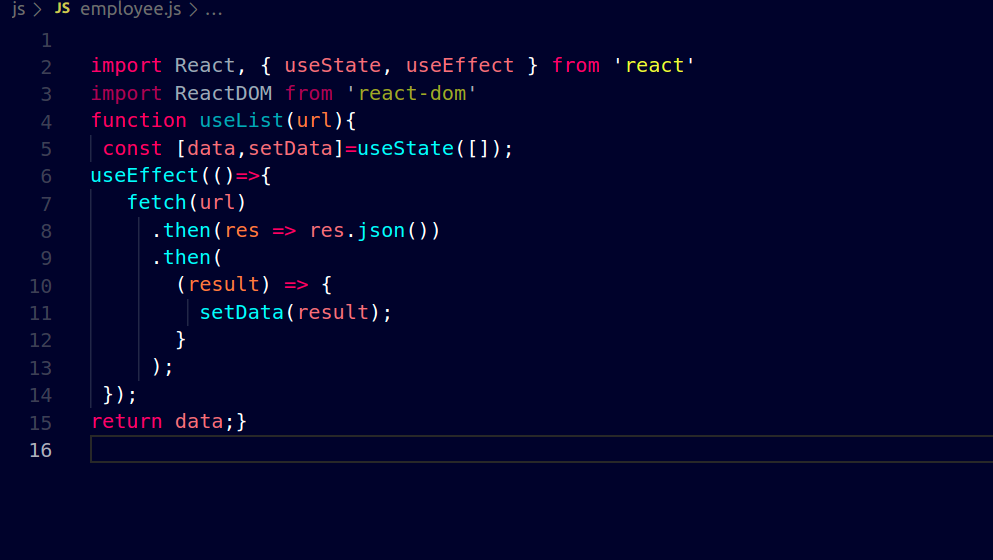
Function Employee():
Employee Component connects to a Web API, fetches the employee data when the Component is mounted, and displays the data.
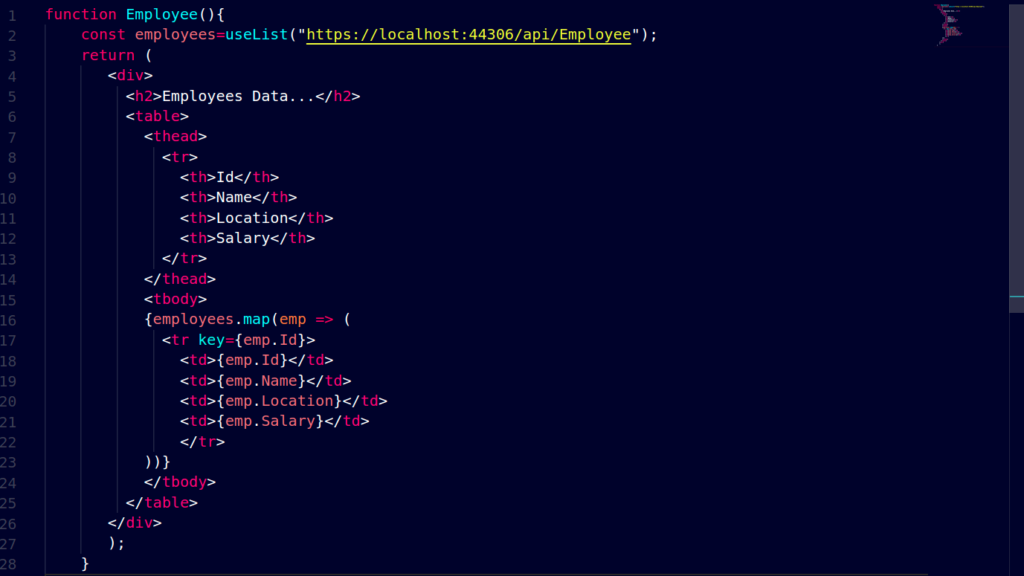
Function Department():
Department Component connects to a Web API, fetches the department’s data when the Component is mounted, and displays the data.
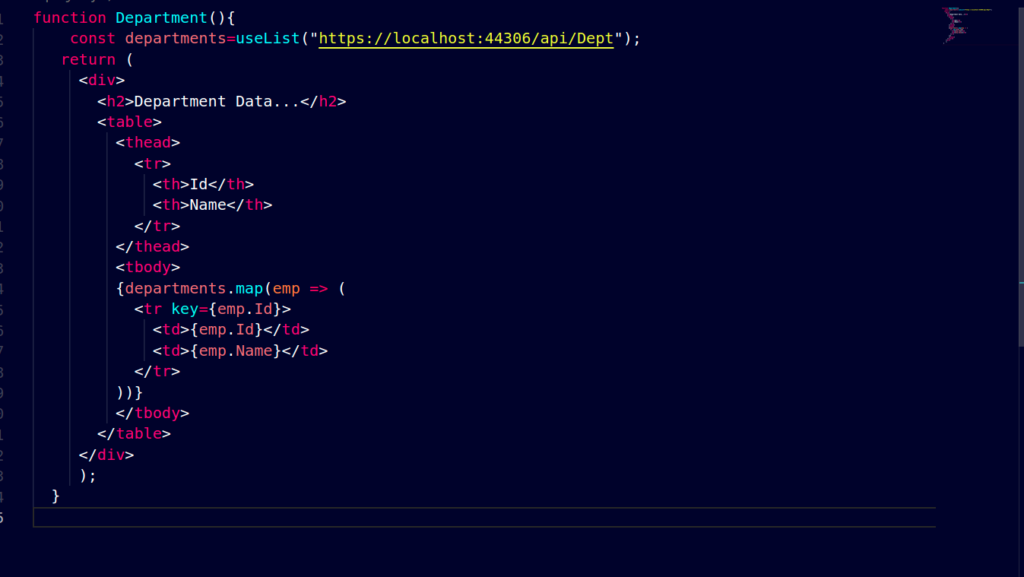
Function App() :
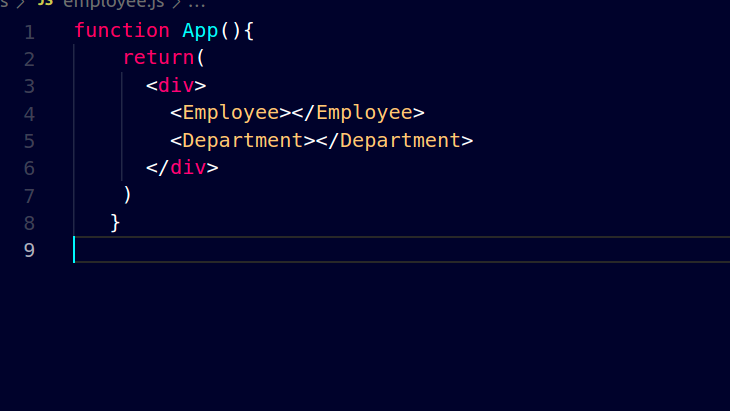
Advantages:
- Sharing logic across various components.
- Being able to extract and reuse logic between components without introducing extra nesting into trees.
- With hooks, we separate code not based on the lifecycle method name but based on what the code is doing.
Disadvantages:
- It’s hard to reuse stateful logic between components.
- Complex components become hard to understand.
Limitations:
- Only call hooks at the top Level
- Only Call hooks from React function components.
Conclusion:
Custom Hooks really opens up new ways to write components, allowing you to tailor the functionality to your liking. Overall, Hooks have added a lot of flexibility to how we can write react apps by minimizing the need for class-based components. You can expand on the functionality of these hooks using some additional Hooks that come built-in with React to create even more amazing Hooks of your own.