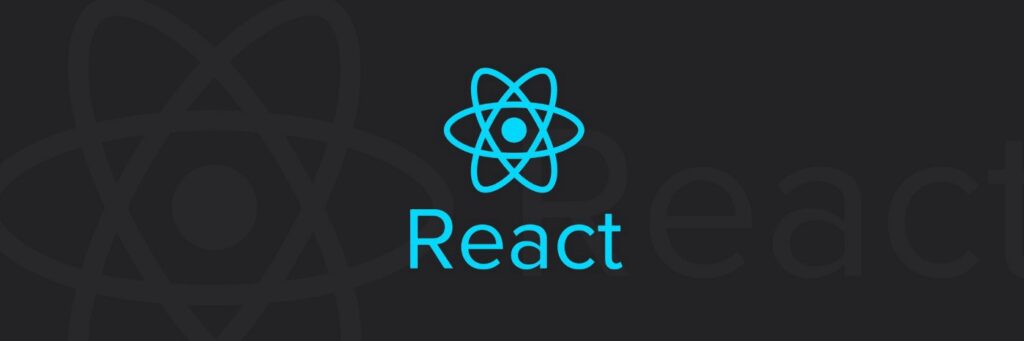
JSX stands for JavaScript Extension which allows us to write javascript function in HTML. The fundamental syntax of JSX is:
React.createElement(component, props, …children) function.
But JSX is not runnable inside today’s browsers. It must be compiled into JavaScript that the browser understands. In React, the library uses a virtual DOM to create an in-memory representation of the DOM. To create these virtual DOM elements, react has a React.createElement function that it calls.
We can use many tools to compile our JavaScript. A popular choice is Babel. There is a transform in Babel, called babel-plugin-transform-react-jsx that does the JSX transform.
Create a react application
Follow the below steps to understand the babel related concept
Step 1: Create a react application using the following command.
import React from ‘react’;import ReactDOM from ‘react-dom’; const ele = ( <div> <h1 id=”h1″> Welcome to Club </h1> <p> Don’t stop learning </p> </div>);ReactDOM.render(ele, document.getElementById(‘root’)); |
Without using JSX: The code that is written in JSX converts into react code using babel compiler as shown below:
import React from ‘react’;
import ReactDOM from ‘react-dom’;
const ele = React.createElement(
“div”, { “class”: “container” },
React.createElement(
“h1”, { id: “h1” }, “Welcome toClub”),
React.createElement(“p”, null, “Don’t stop learning”));
ReactDOM.render(ele, document.getElementById(‘root’));
React Uses a Virtual DOM
React works differently than many earlier front-end JavaScript frameworks in that instead of working with the browser’s DOM, it builds a virtual representation of the DOM. By virtual, we mean a tree of JavaScript objects that represent the “actual DOM”. More on this in a minute.
In React, we do not directly manipulate the actual DOM. Instead, we must manipulate the virtual representation and let React take care of changing the browser’s DOM.
Why Not Modify the Actual DOM?
It’s worth asking: why do we need a Virtual DOM? Can’t we just use the “actual-DOM”?
When we do “classic-” (e.g. jQuery-) style web development, we would typically:
- locate an element (using document.querySelector or document.getElementById) and then
- modify that element directly (say, by setting .innerHTML on the element).
This style of development is problematic in that:
- It’s hard to keep track of changes – it can become difficult to keep track of current (and prior) state of the DOM to manipulate it into the form we need.
- It can be slow – modifying the actual-DOM is a costly operation, and modifying the DOM on every change can cause significantly degrade the performance.
What is a Virtual DOM?
The Virtual DOM was created to deal with these issues. But what is the Virtual DOM anyway?
The Virtual DOM is a tree of JavaScript objects that represents the actual DOM.
One of the interesting reasons to use the Virtual DOM is the API it gives us. When using the Virtual DOM we code as if we’re recreating the entire DOM on every update.
This idea of re-creating the entire DOM results in an easy-to-comprehend development model: instead of the developer keeping track of all DOM state changes, the developer simply returns the DOM they wish to see. React takes care of the transformation behind the scenes.
This idea of re-creating the Virtual DOM every update might sound like a bad idea: isn’t it going to be slow? In fact, React’s Virtual DOM implementation comes with important performance optimizations that make it very fast.
The Virtual DOM will:
- use efficient diffing algorithms, in order to know what changed
- update subtrees of the DOM simultaneously
- batch updates to the DOM