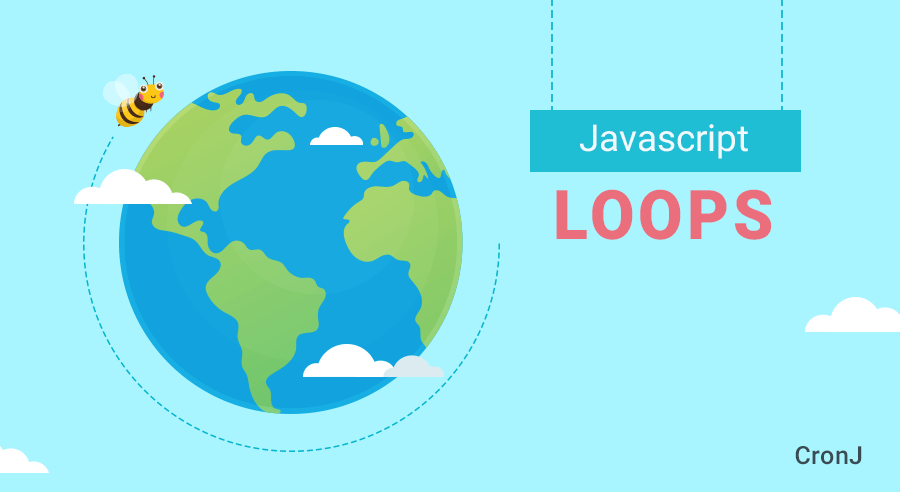
Loops in JavaScript
JavaScript loops provide a quick and easy method of doing something repeatedly. They are used to repeat an action number of times without having to repeat the same line of code.
There are mainly two types of loops:
- Entry Controlled Loops – In entry controlled, the test condition is tested before entering the loop body. For Loop and While Loop are entry-controlled loops.
- Exit Controlled Loops– In exit controlled, the test condition is evaluated at the end of the loop body. Therefore, the loop body will execute at least once, irrespective of whether the test condition is true or false. do-while loop is an exit controlled loop.
For Loop
This method provides a concise way of writing the loop structure. A for loop repeats until a specified condition evaluates to false.
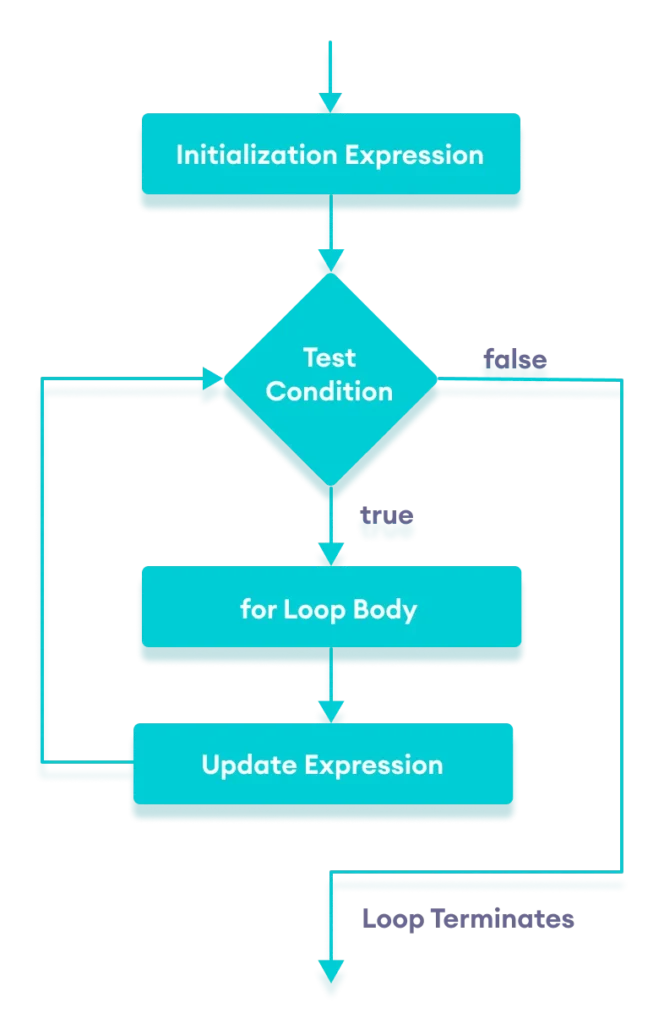
Syntax:
for (initialization condition; testing condition; increment/decrement)
{
statement
}
Example:
<script type = “text/javaScript”>
var x;
for (x = 1; x <= 5; x++)
{
document.write(“Value of x:” + x + “<br />”);
}
< /script>
Output-
Value of x:1
Value of x:2
Value of x:3
Value of x:4
While Loop:
A while loop is a control flow statement that allows the code to be executed repeatedly based on a particular Boolean condition. This loop is similar to that of a repeating if statement.
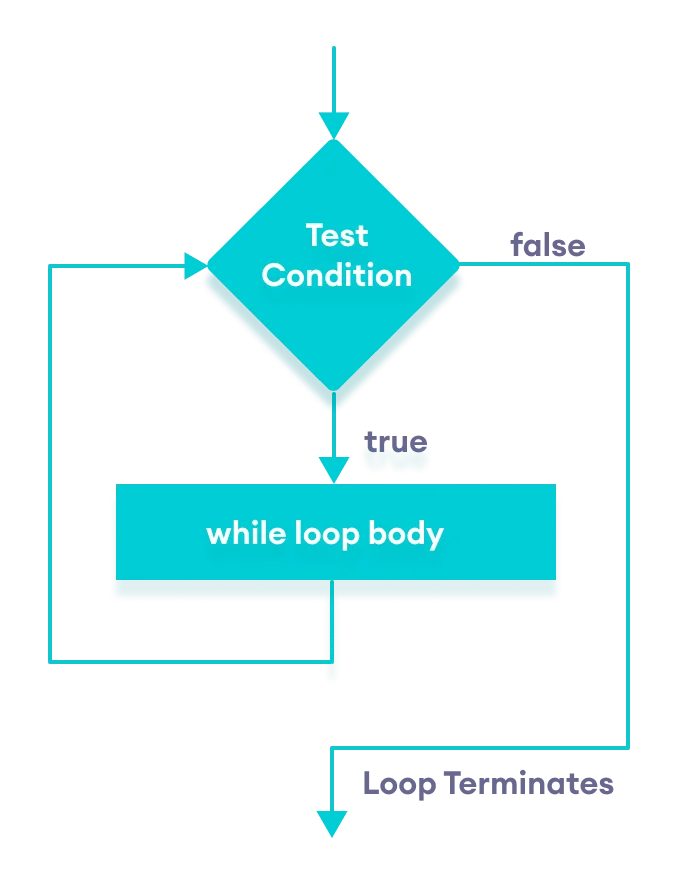
Syntax:
while (boolean condition)
{
loop statements
}
Example:
<script type = “text/javaScript”>
var x = 1;
while (x <= 5){
document.write(“Value of x:” + x + “<br />”);
x++;}
< /script>
Output: Value of x:1 Value of x:2 Value of x:3 Value of x:4 Value of x:5
Do-while Loop: This loop is similar to while loop but the only difference is that it checks for condition after executing the statements. Thus, it is an example of Exit Controlled Loop. Syntax: do { statements } while (condition); Example: <script type = "text/javaScript"> var x = 20; do { document.write("Value of x:" + x + "<br />"); x++; } while (x < 10); </script> Output: Value of x: 20 For-in Loop: This loop iterates a specified variable over all the enumerable properties of an object. For each distinct property, JavaScript will execute the specified statements. Syntax: for (variableName in Object) { statements } Example: <script type = "text/javaScript"> var characteristic = { first : "Name", second : "Age", third : "Height", fourth : "Eye-color", fifth : "Nationality" }; for (itr in characteristics) { document.write(characteristics[itr] + "<br >"); } </script> Output: Name Age Height Eye-color Nationality Break Statement: The break statement is used for jumping out of a loop. It will help you in breaking the loop and continue executing the code after the loop. Syntax: break labelname; Example: var text = "" var i; for (i = 0; i < 10; i++) { if (i === 5) { break; } text += "The number is " + i + "<br>"; } Output: The number is 0 The number is 1 The number is 2 The number is 3 The number is 4 Continue Statement: The continue statement breaks one iteration in the loop if a specified condition occurs, and continues with the next iteration in the loop. The difference between continue and the break statement is that the continue statement “jumps over” one iteration in the loop instead of “jumping out”. Syntax: continue labelname; Example: var text = "" var i; for (i = 0; i < 5; i++) { if (i === 2) { continue; } text += "The number is " + i + "<br>"; } Output: The number is 0 The number is 1 The number is 3 The number is 4 References: https://www.w3schools.com/ https://www.javascript.com/ https://www.edureka.co/blog/javascript-tutorial/