In React, you may have seen most of the times the application crashed before render and caused a crashed UI with some error on the screen. You can say while loading app, crashed during render phase corrupting react state internally.
So Js error occurred during the render, React didn’t provide a way to handle those error causing applications with crashed UI. It’s inescapable that we’ll encounter unexpected errors in our apps. You could be trying to access a deeply-nested property on an object that doesn’t exist, or sometimes it’s not in your control (like a failed HTTP request to a 3rd-party API).
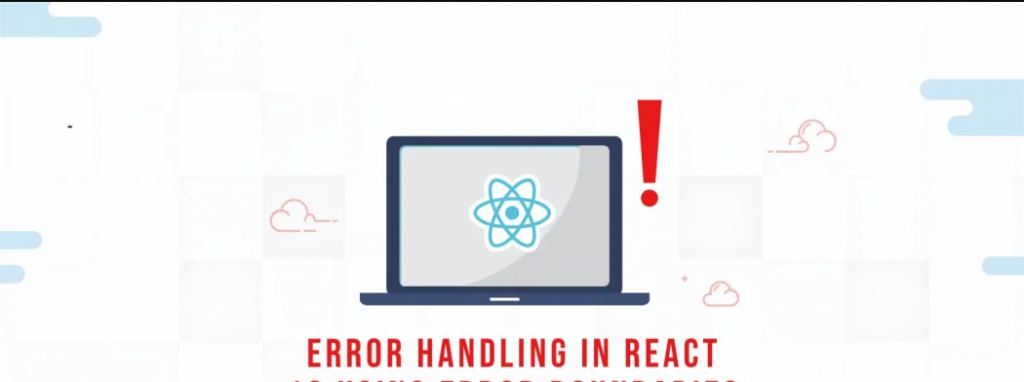
Application without Error Boundary:-
Without Error Boundaries in the application, it will cause an unavoidable error in the initial render of application.
In the demo below, We will simulate the error and will find out what happens without error boundary.

Output:
When the application encounters an error showing with the crashed UI with an error message, technically it doesn’t look good for our application or to the user.
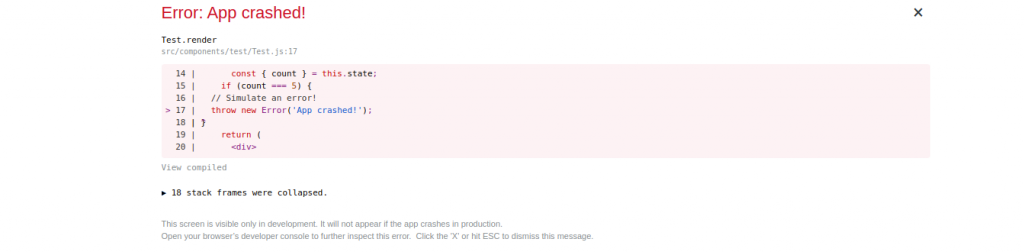
Application With Error Boundary:-
So we will use the Error Boundary of React. Error Boundary is the react component that helps in handling the error which is introduced by React16. It will find out the js error throughout the application including its child tree components and display fallback UI.
In other words, it’s more like a strategy of handling errors in React during render.
Specifically, it’s the usage of two lifecycle methods of React i.e
- Static getDerivedStateFromError()
- componentDidCatch()
Below Code demo will show how we implement ErrorBoundary in React.
- Create a new file. Eg: MyErrorBoundary.
- Add the Error Boundary code to the file.
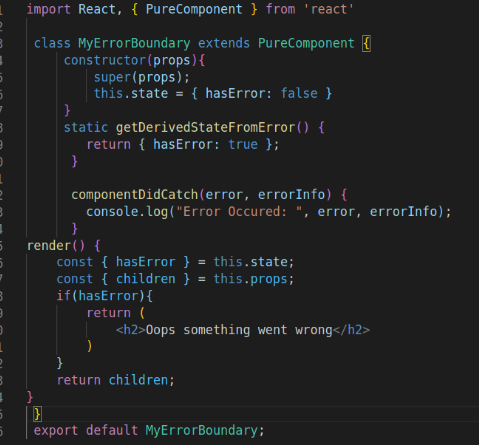
As the above code has two lifecycle methods which explain:
Static getDerivedStateFromError() — It renders the fallback UI that we have created on simulating error.
componentDidCatch()- It will contain two arguments to log the error and showing the information which will help to debug the error.
So what is an Error Boundary? Any React Component is considered an Error Boundary when it employs at least one of these lifecycle methods.
For displaying fallback UI you have to wrap our component with MyErrorBoundary in App.js
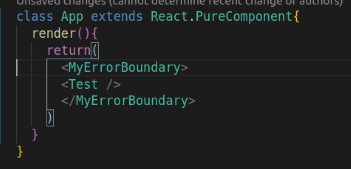
Output:
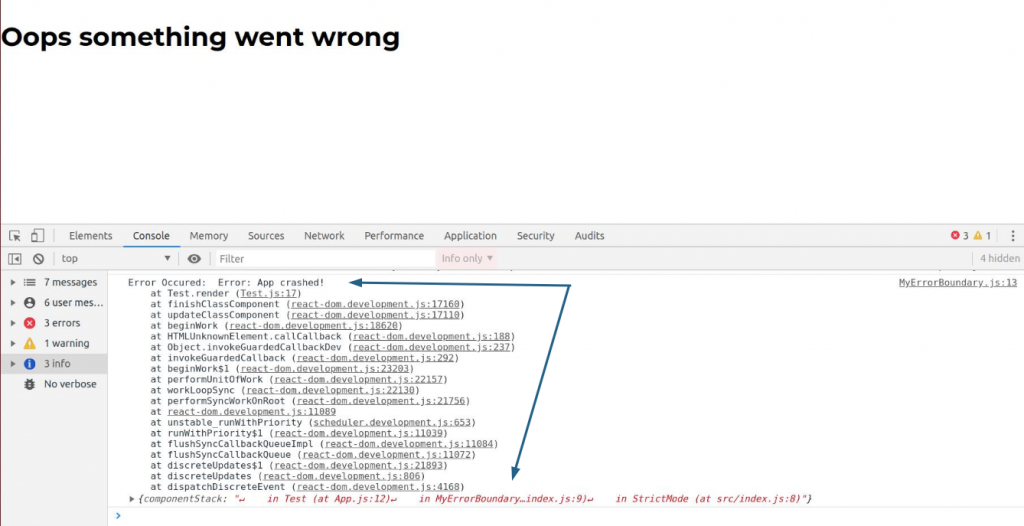
Where we can Place Error Boundaries?
- We can wrap our application in ErrorBoundary to topmost levels of route components.
- We can wrap individual components.
Some Important points to note:-
Error boundary could only intercept errors originating from :
- In Lifecycle methods.
- During render.
- In the constructor.
They won’t work with?
- Event handlers.
- setTimeout.
- requestAnimationFramecallback.
- Error caused itself in Error Boundary.
- Server-side rendering(SSR).
- Only class components can have an error boundary.
Which again raises the question of why we can’t use an Error Boundary with a functional component??
- Working with hooks to ensure an error boundary will be very much trickier and recommended not to use. Which means we can use error boundary with our functional components but with the major consequences.
- If we really want to use Error Boundary with our functional components then we have two ways i.e.
- Wrapping our functional component with the class component
- Or we can use a third-party package for the same.
In conclusion, It’s better to have user readability with a nice UI fallback instead of a crashed screen code screen.
Thanks for scrolling this far. I hope you liked the article.
Reference: https://reactjs.org