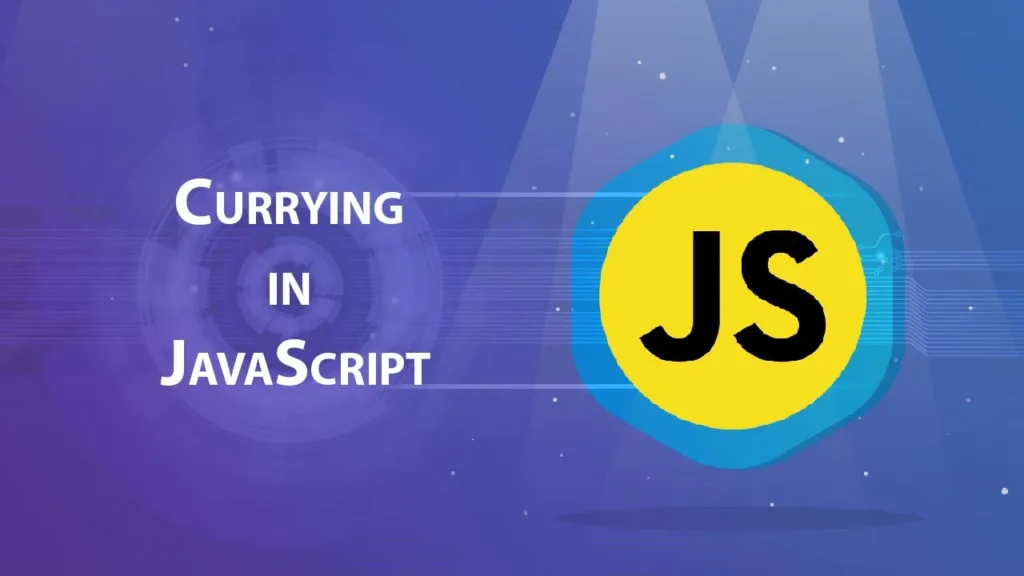
Currying simply means evaluating functions with multiple arguments and decomposing them into a sequence of functions with a single argument.
In other terms, currying is when a function — instead of taking all arguments at one time — takes the first one and returns a new function, which takes the second one and returns a new function, which takes the third one, etc. until all arguments are completed.
An American mathematician named Haskell curry developed this technique, that’s why it is called Currying.
Why should I use currying?
There are several reasons why currying is ideal:
- Currying is a checking method to make sure that you get everything you need before you proceed
- It helps you to avoid passing the same variable again and again
- It divides your function into multiple smaller functions that can handle one responsibility. This makes your function pure and less prone to errors and side effects
- It is used in functional programming to create a higher-order function
- This could be a personal preference, but I love that it makes my code readable
How does currying work?
Currying is a function that accepts multiple arguments. It will transform this function into a series of functions, where every little function will accept one argument
//Noncurried version
const add = (a, b, c)=>{
return a+ b + c
}
console.log(add(2, 3, 5)) // 10
//Curried version
const addCurry =(a) => {
return (b)=>{
return (c)=>{
return a+b+c
}
}
}
console.log(addCurry(2)(3)(5)) // 10
Creating a friend request curry function:
In this example, we are going to create a simple curry function where a user sends a friend request to his friend Vijay::
function sendRequest(greet){
return function(name){
return function(message){
return `${greet} ${name}, ${message}`
}
}
}
sendRequest('Hello')('Vijay')('Please can you add me to your Instagram Followers?');
Output:
“Hello Vijay, Please can you add me to your Instagram Followers?”
We created a function sendRequest that requires only one argument, greet, and it returns the name of the person and the message we want to send to the user. Then, when we invoked the function, it outputted the message.
Conclusion:
Closure makes currying possible in Javascript. It’s the ability to retain the state of functions already executed and gives us the ability to create factory functions- functions that can add a specific value to their argument.
References:
https://javascript.info/currying-partials
https://www.geeksforgeeks.org/what-is-currying-function-in-javascript/