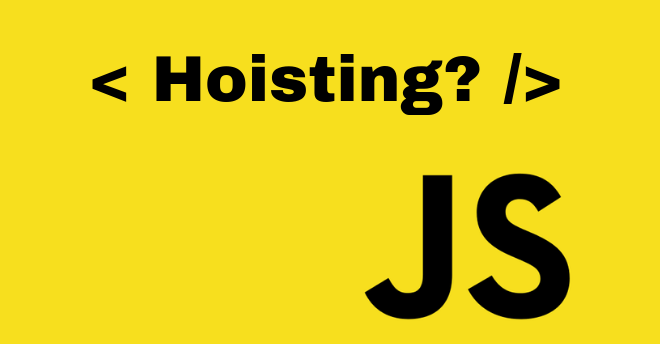
Hoisting –
- Hoisting in JavaScript is a behavior in which a function or a variable can be used before declaration. For example,
a=5;
Var a ;
Console.log(a) // result will be 5
Variable Hoisting – Here we declare then initialize the value of a var after using it. The default initialization of the var is undefined.
console.log(num); // Returns ‘undefined’ from hoisted var declaration (not 1)
var num; // Declaration
num = 1; // Initialization
console.log(num); // Returns 1 after the line with initialization is executed
The same thing happens if we declare and initialize the variable in the same line.
console.log(num); // Returns ‘undefined’ from hoisted var declaration (not 1)
var num = 1; // Initialization and declaration.
console.log(num); // Returns 1 after the line with initialization is executed.
let and const Hoisting – Variables declared with let and const are also hoisted but, unlike var, are not initialized with a default value. An exception will be thrown if a variable declared with let or const is read before it is initialized.
console.log(num); // Throws ReferenceError exception as the variable value is uninitialized
let num = 1; // Initialization
For information and examples see let > temporal dead zone
Temporal dead zone (TDZ)
let variables cannot be read/written until they have been declared. If no initial value is specified on declaration, the variable is initialized with a value of undefined. Accessing the variable before the declaration results in Reference Error.
The variable is said to be in a “temporal dead zone” (TDZ) from the start of the block until the declaration has completed.
{ // TDZ starts at beginning of scope
console.log(b); // undefined
console.log(foo); // ReferenceError
var b = 10;
let a = 20; // End of TDZ (for a)
}
The term “temporal” is used because the zone depends on the order of execution (time) rather than the order in which the code is written (position). For example, the code below works because, even though the function that uses the let variable appears before the variable is declared, the function is called outside the TDZ.
{
// TDZ starts at beginning of scope
const func = () => console.log(letVar); // OK
// Within the TDZ letVar access throws `ReferenceError`
let letVar = 3; // End of TDZ (for letVar)
func(); // Called outside TDZ!
}
Event Bubbling –
- Event bubbling is a method of event propagation in the HTML DOM API when an event is in an element inside another element, and both elements have registered a handle to that event. It is a process that starts with the element that triggered the event and then bubbles up to the containing elements in the hierarchy. In event bubbling, the event is first captured and handled by the innermost element and then propagated to outer elements.
Example of Event Bubbling:
Let’s look at the below example to understand the working concept of Event Bubbling:
<!DOCTYPE html>
<html>
<head>
<meta charset=”utf-8″>
<meta name=”viewport” content=”width=device-width”>
<title>Event Bubbling</title>
</head>
<body>
<div id=”d1″>
<button id=”b1″>I am child button</button>
</div>
<script>
var parent = document.querySelector(‘#d1’);
parent.addEventListener(‘click’, function(){
console.log(“Parent is invoked”);
});
var child = document.querySelector(‘#b1’);
child.addEventListener(‘click’, function(){
console.log(“Child is invoked”);
});
</script>
</body>
</html>
- Now , Understand above code to understand Event Bubbling——————-
- In the Above HTML javascript code ,
- We have used a div tag having div id = d1 and within div we have nested a button having button id = b1. Now, within the JavaScript section, we have assigned the HTML elements (d1 and b1) using the querySelector () function to the variable parent and child.
- After that, we have created and included an event which is the click event to both the div element and child button. Also created two functions that will help us to know the sequence order of the execution of the parent and child. It means if the child event is invoked first, “child is invoked” will be printed otherwise “parent is invoked” will get printed.
- Thus, when the button is clicked, it will first print “child is invoked” which means that the function within the child event handler executes first. Then it moves to the invocation of the div parent function.
The sequence has taken place due to the concept of event bubbling.
Document
?
Body
?
Html
?
Div
?
Button
It means when the user click on the button, the click event flows in this order from bottom to top.