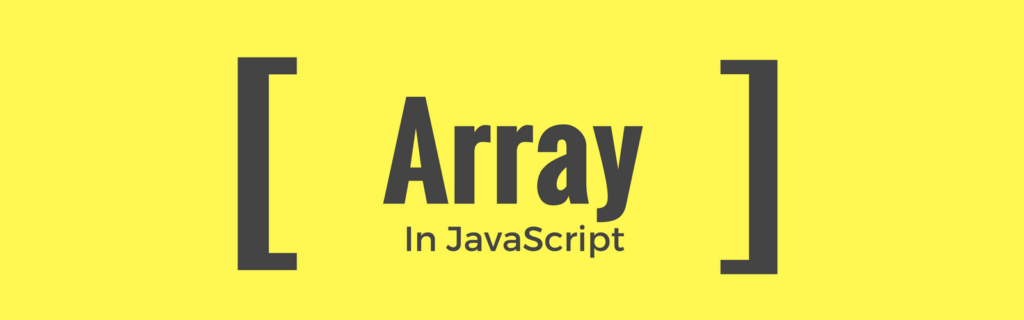
What is an array?
An array is a special variable, which can hold more than one value.
Syntax: const array_name = [item1, item2, …];
Example: const cars = [“Saab”, “Volvo”, “BMW”];
There are 3 ways to construct array in JavaScript
1)By array literal:
Example: const cars = [“Saab”, “Volvo”, “BMW”];
2) By creating instance of Array directly (using new keyword)
Example: var emp = new Array();
- emp[0] = “Arun”;
- emp[1] = “Varun”;
- emp[2] = “John”;
Array Methods:
concat() –
The concat() method creates a new array by concatenating or merging existing arrays. It does not modify the existing array and always returns a new array.
Example:
var arr1=[“Vijay”,”jadhav”];
var arr2=[“Kashi”];
var result=arr1.concat(arr2);
document.writeln(result);
output:vijay,jadhav,Kashi
push() –
It is easy to remove elements and add new elements while working with arrays. The push() method adds a new element to the end of an array. The return value is the new array length.
Example:
let arr=[1,2,3,4,5];
arr.push(10)
document.write(arr)
o/p: 1,2,3,4,5,10
pop() –
The pop() method is used to remove the last element from an array. It returns the value that has been popped out.
Example:
let arr=[1,2,3,4,5];
arr.pop()
document.write(arr)
o/p:1,2,3,4
shift() –
Shifting is similar to popping, working on the first element instead of the last. The shift() method is used to remove the first array element and shifts all other elements to a lower index. It will return you the string that has been shifted out.
Example:
let arr=[1,2,3,4,5];
arr.shift()
document.write(arr)
o/p:2,3,4,5
unshift() –
This method adds a new element at the beginning of an array and unshifts older elements. It is similar to Push() and returns the new array length.
Example:
let arr=[1,2,3,4,5];
arr.unshift(20)
document.write(arr)
output:20,1,2,3,4,5
toString() –
The toString() method is used to convert an array to a string of array values, separated by commas.
Example:
let arr=[1,2,3,4,5];
let arr2=arr.toString()
document.write(typeof(arr2[0]))
o/p:String
join() –
The join() method works same as toString(). It is used to join all array elements into a string, but in addition, you can specify the separator.
Example:
let arr=[1,2,3,4,5];
let arr2=arr.join(“+”)
document.write(arr2)
op:1+2+3+4+5
reverse() –
It returns the element of the given array in a sorted order.
Example:
let arr=[“kashi”,”jadh”];
let arr2=arr.reverse()
document.write(arr2)
o/p: jadh,kashi
slice() –
The slice() method is used to slice out a piece of an array into a new array. It creates a new array without removing any elements from the source array. It will return the value that has been sliced out from the array.
Example:
let arr=[‘a’,’b’,’c’,’d’,’e’,’f’,’g’]
let arr2=arr.slice(1,4)
document.write(arr2)
o:p: b,c,d,e
map() –
It calls the specified function for every array element and returns the new array.
Example:
let arr=[1,2,3,4,5]
let arr2=arr.map(multiply)
function multiply(value,index,array)
{
return value*2;
}
forEach():
It invokes the provided function once for each element of an array.
Example:
let arr=[1,2,3,4,5]
arr.forEach(multiply)
function multiply(item,index,array)
{
document.write(“at “,index ,” index “,item, “<br>”)
}
output
at 0 index 1
at 1 index 2
at 2 index 3
at 3 index 4
at 4 index 5
filter()–
It returns the new array containing the elements that pass the provided function conditions.
Example:
let arr=[1,2,3,4,5,6]
document.write(arr.filter(checkEven))
function checkEven(item,index,array)
{
if(item%2==0)
{
return true;
}
else
{
return false;
}
}
reduce() –
It executes a provided function for each value from left to right and reduces the array to a single value.
Example:
let arr=[1,2,3,4,5];
document.write(arr.reduce(addition,0))
function addition(initial,value)
{
return initial+value;
}
o:p: 15
splice():
It add/remove elements to/from the given array.
Example:
let arr=[10,11,12,13,14,15]
arr.splice(2,2,”kashi”,”jadhav”) //first parameter is starting index,second is how many element wants to delete and third is optional i.e. add element
document.write(arr)
o/P: 10,11,kashi,jadhav,14,15
fill():
It fills elements into an array with static values.
Example:
let arr=[1,2,3,4,5]
document.write(arr.fill(0))
o/p:0,0,0,0,0
indexOf():
It searches the specified element in the given array and returns the index of the first match.
Example:
let arr=[10,12,13,11];
document.write(arr.indexOf(12))
o/p:1
includes():
It checks whether the given array contains the specified element.
Example:
let arr=[1,2,3,4]
document.write(arr.includes(32))
o/p:false
flat():
It creates a new array carrying sub-array elements concatenated recursively till the specified depth.
example:
let arr=[1,2,3,[,[5,6,7]]]
document.write(arr.flat())
output: 1,2,3,4,5,6,7
every() –
It determines whether all the elements of an array are satisfying the provided function conditions.
example:
let arr=[2,4,6,7]
document.write(arr.every(EvenNumber))
function EvenNumber(item,index)
{
return item%2==0
}
o/p:false
some() –
It determines if any element of the array passes the test of the implemented function.
example:
let arr=[11,11,22]
document.write(arr.some(evenNumber))
function evenNumber(item,index)
{
return item%2==0
}
o/p:False
References:
https://www.w3schools.com/js/js_arrays.asp
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array